Creating an HTTP API model
-
In Polytomic, go to Models → Add Model → Select your HTTP connection.
-
Name your API model. It is a good idea to name your model after the entities it imports from your API.
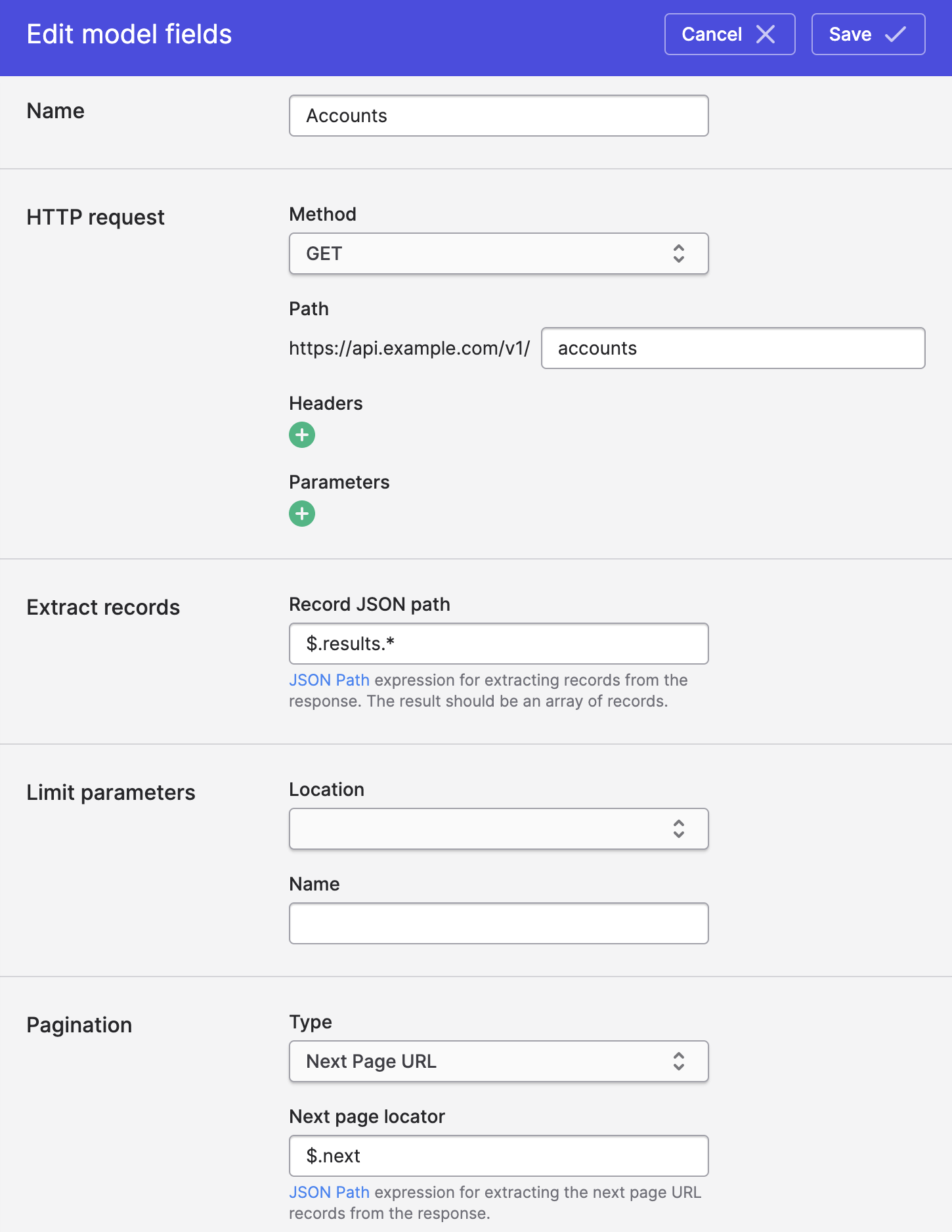
-
Select GET or POST for the method body.
-
Enter the path and any required headers and query parameters for the request.
-
You can click the Refresh button below to see the API response based on your configuration.
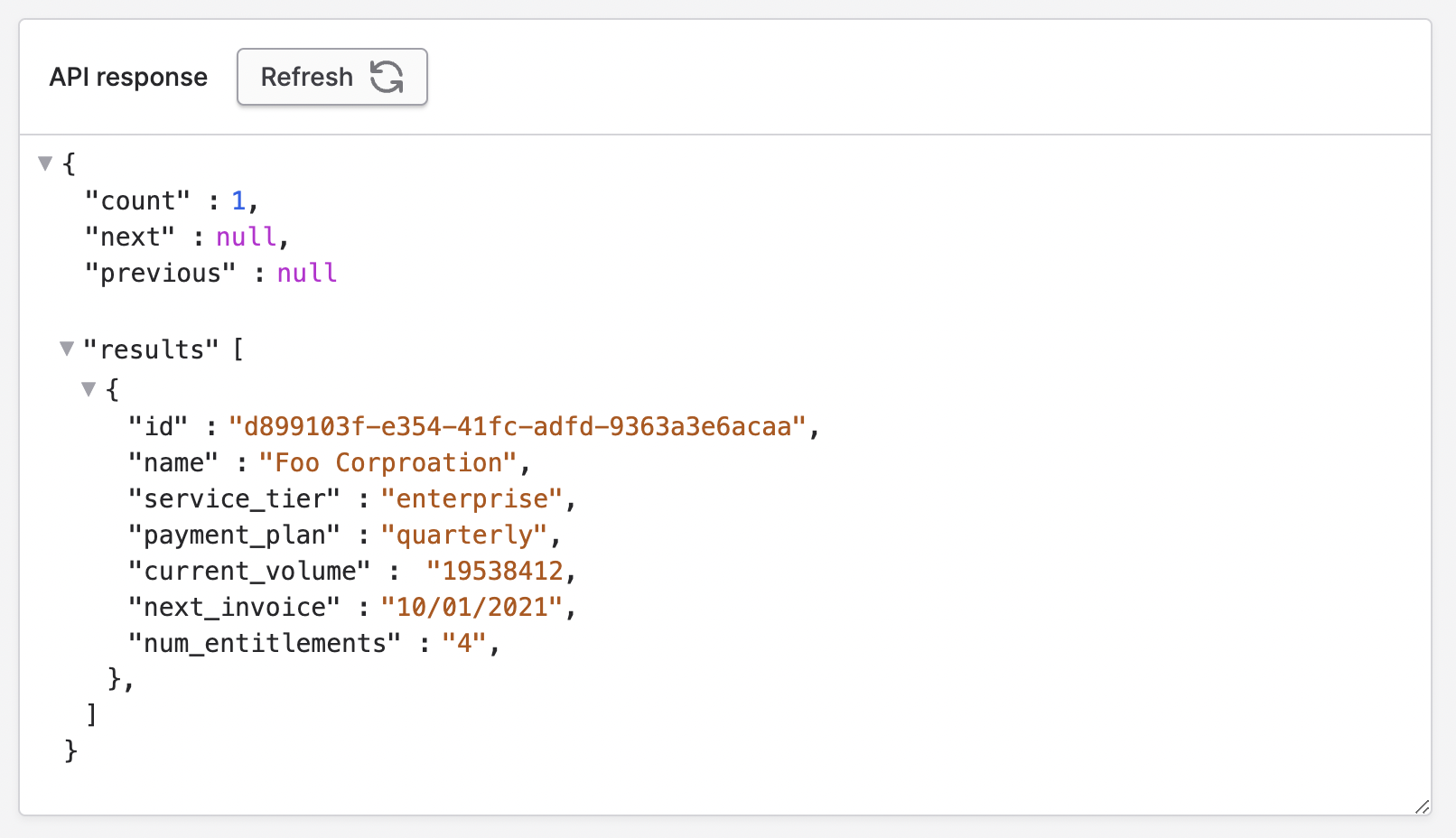
-
You need to instruct Polytomic how to extract the records from the JSON response. Insert a JSONPath expression into the Extract records field to specify your array of records within the API response. You can learn more https://docs.polytomic.com/docs/using-json-path-for-model-creation, which has some examples as well as links to further reading.
-
After entering the record-extraction JSON Path expression, click Refresh again and you'll see a list of model fields. Select those you want to surface in Polytomic.
-
Commonly, HTTP APIs provide only a subset of the total results at a time. Polytomic can paginate through the results automatically if you specify your API's pagination method. There are four options:
- Next Page URL: enter the JSON Path expression for finding the next page URL in the response body.
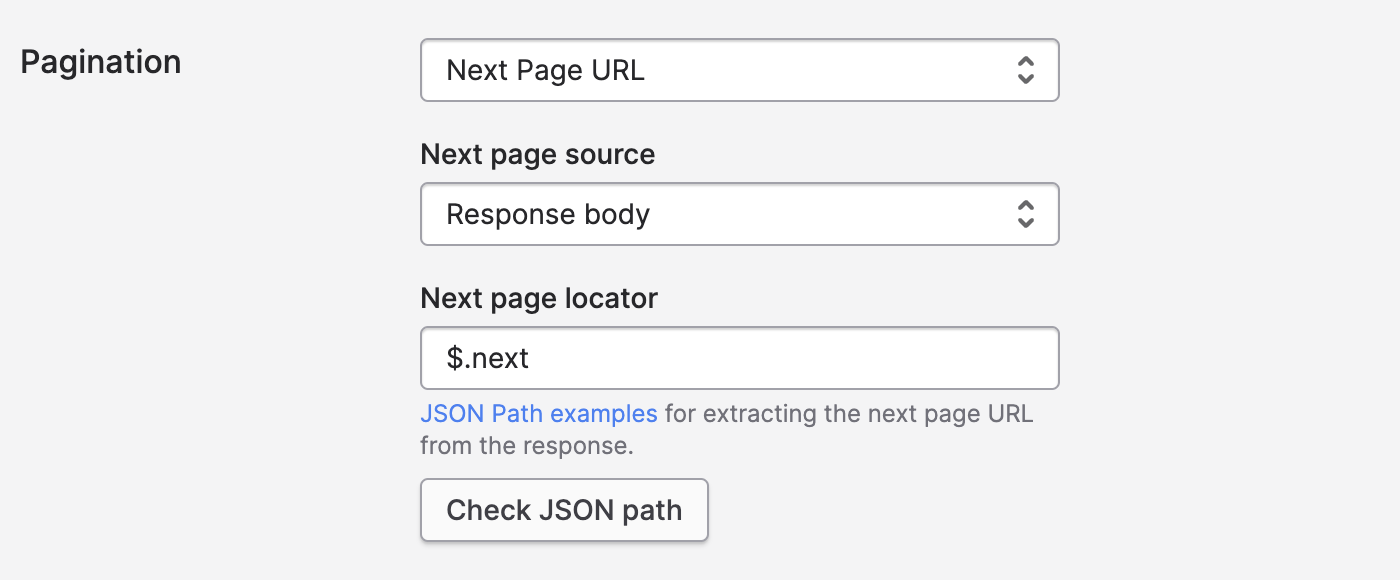
- Token: if the response has a token to be included in a header or query parameter, use JSONPath to extract it and specify the field name-value pair as either a header or query parameter.
- Sequential page: if there is a query parameter to indicate the page number being accessed
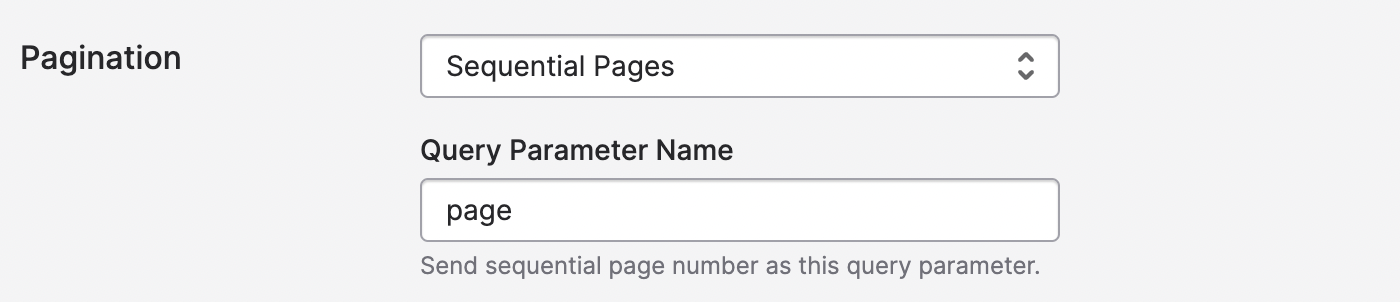
- Offset: if there is a query parameter to indicate the first result number being accessed. You will also need to provide who many results can be accessed per page, and optionally a query parameter to specify the number of results being queried per page.
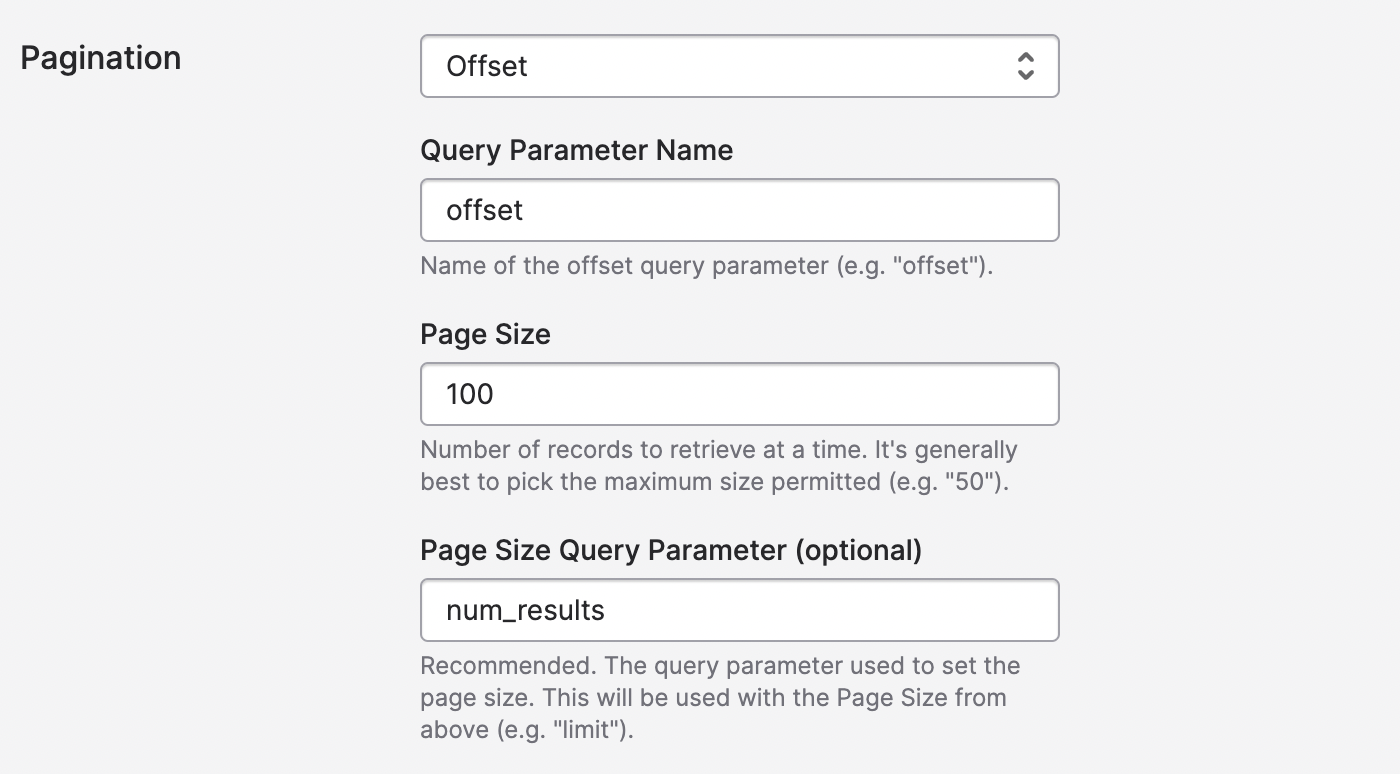
-
(optional) Join this model to another of your models.
-
Click Save.
Datetime Expressions in Headers and Query Parameters
You may find it necessary to send dynamic datetime values in headers or parameters. An example of this is wanting to send "ten days ago, from the time the sync runs" as a parameter. To support this, Polytomic allows you to write simple datetime expressions in the model Headers and Parameters fields.
Here is an example expression that allows sending a startDate
query parameter that will evaluate to a week ago:
{{ add_days(-7, now()) }}
Token Expressions
You may use an expression to modify the pagination token before it is sent. The pagination token is available in the expression as token()
.
For example, if you wanted to trim timezone information from the token, you could do so with an expression like so: {{ first_n_chars(token(), 19) }}
.
Expression Manual
Types
Date
types will render by default as "YYYY-MM-DD".
Datetime
types will render by default as "YYYY-MM-DDTHH:MM:SSZ"
Date
and Datetime
can usually be used interchangeably. However, if you add hours/minutes/seconds to a Date, it will be cast to a Datetime.
Function | Parameters/Return | Notes |
---|---|---|
now() | Returns: Datetime Object representing the current date/time in UTC. | Returns current time in UTC. |
date(year int, month int, day int) | Param year Param month : month expressed numerically. January is 1 .Param day : day of month. The first day is 1 .Returns: Date Object | |
date_time(year int, month int, day int, hour int, minute int, second int) | Year/Month/Day parameters as above. Param hour : hour of day, beginning at "0".Param minute : minute of hour, beginning at "0".Param second : second of minute, beginning at "0".Returns: Datetime Object | Datetimes are returned in UTC values only. |
extract_years(datetime DateTime) | - Param datetime : date to get the year of- Returns: int representing year | |
extract_months(datetime Datetime) | - Param datetime : date to get the month of- Returns: int representing the month. (January is 1 ). | |
extract_days(datetime Datetime) | - Param datetime : date to get the days of- Returns: int representing the day. The first day of the month is 1 . | |
extract_hours(datetime Datetime) | - Param datetime : date to get the hours of- Returns: int representing the hour of the day. The first hour of the day is 0 . | Time component will be provided in UTC. |
extract_minutes(datetime Datetime) | - Param datetime : date to get the minutes of- Returns: int representing the minute of the hour. | Time component will be provided in UTC. |
extract_seconds(datetime Datetime) | - Param datetime : date to get the seconds of- Returns: int representing the seconds of the minute. | |
add_years(years_to_add int, datetime Datetime) | - Param years_to_add : how many years to add- Param datetime : Datetime to add years to- Returns: Datetime with specified years added to it | Negative values are permitted if you would like to specify a time in the past. |
add_months(months_to_add int, datetime Datetime) | - Param months_to_add : how many months to add- Param datetime : Datetime to add months to- Returns: Datetime with specified months added to it | Negative values are permitted if you would like to specify a time in the past. |
add_days(days_to_add int, datetime Datetime) | - Param days_to_add : how many days to add- Param datetime : Datetime to add days to- Returns: Datetime with specified days added to it | Negative values are permitted if you would like to specify a time in the past. |
add_hours(hours_to_add int, datetime Datetime) | - Param hours_to_add : how many hours to add- Param datetime : Datetime to add hours to- Returns: Datetime with specified hours added to it | Negative values are permitted if you would like to specify a time in the past. |
add_minutes(minutes_to_add int, datetime Datetime) | - Param minutes_to_add : how many minutes to add- Param datetime : Datetime to add minutes to- Returns: Datetime with specified minutes added to it | Negative values are permitted if you would like to specify a time in the past. |
add_seconds(seconds_to_add int, datetime Datetime) | - Param seconds_to_add : how many seconds to add- Param datetime : Datetime to add seconds to- Returns: Datetime with specified seconds added to it | Negative values are permitted if you would like to specify a time in the past. |
datetime_to_ms(datetime Datetime) | - Param datetime : Datetime to convert to milliseconds- Returns: int converted datetime | You can perform basic math on this number. For example, you can send microseconds or nanoseconds by multiplying. e.g. {{ datetime_to_ms(now()) * 1000 }} |
format_datetime(datetime Datetime, layout string) | - Param datetime : Datetime to format- Param layout : a pattern representing how to format a Datetime- Returns: string date formatted according to layout | The layout pattern should be Go-compatible Time layout specification. |
first_n_chars(input string, nChars int) | - Param input : the string to take the first characters of- Param nChars : how many characters to preserve- Returns The first nChars of input . |
Updated almost 3 years ago